Introduction:
React is known for its high performance, but as your application grows, you may encounter performance issues. One way to improve performance is by using memoization techniques like useMemo and useCallback. In this blog post, we’ll explore how to use useMemo and useCallback in a Counter component to improve performance.
Simple Counter Component in React:
Let’s consider a simple Counter component that increments a counter when a button is clicked. The Counter component also has a child component that displays the current count.
import React, { useState } from 'react';
import DisplayCount from './DisplayCount';
function Counter() {
const [count, setCount] = useState(0);
const increment = () => {
setCount(count + 1);
};
return (
<div>
<button onClick={increment}>Increment</button>
<DisplayCount count={count} />
</div>
);
}
export default Counter;
The Problem with Re-rendering: In this component, every time the increment function is called, the count state is updated, and the entire Counter component re-renders, including the child DisplayCount component. This can cause unnecessary re-renders and hurt performance.
Here is the solution to stop re-rendering:
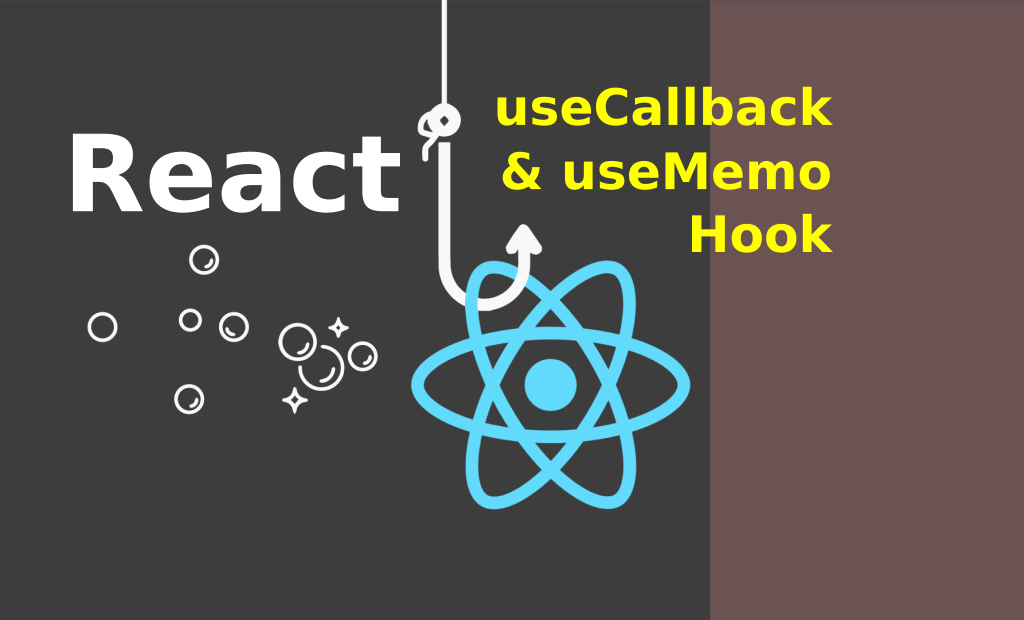
Using useMemo and useCallback: To prevent unnecessary re-renders of the child DisplayCount component, we can use either useMemo or useCallback. Let’s explore how to implement both techniques for the Counter component.
Using useMemo:
import React, { useState, useMemo } from 'react';
import DisplayCount from './DisplayCount';
function Counter() {
const [count, setCount] = useState(0);
const increment = () => {
setCount(count + 1);
};
const memoizedCount = useMemo(() => count, [count]);
return (
<div>
<button onClick={increment}>Increment</button>
<DisplayCount count={memoizedCount} />
</div>
);
}
export default Counter;
In this example, we’re using useMemo to memoize the count value. The second argument of useMemo is an array of dependencies. In this case, the only dependency is the count value, so the memoizedCount value is only updated when the count value changes.
Using useCallback:
import React, { useState, useCallback } from 'react';
import DisplayCount from './DisplayCount';
function Counter() {
const [count, setCount] = useState(0);
const increment = useCallback(() => {
setCount(count + 1);
}, [count]);
return (
<div>
<button onClick={increment}>Increment</button>
<DisplayCount count={count} />
</div>
);
}
export default Counter;
In this example, we’re using useCallback to memoize the increment function. The second argument of useCallback is also an array of dependencies. In this case, the only dependency is the count value, so the increment function is only recreated when the count value changes.
The Benefits of using useMemo and useCallback :
In both cases, the child DisplayCount component will only re-render when the count value changes, preventing unnecessary re-renders and improving performance. By using these memoization techniques, you can improve the performance of your React application.
Conclusion:
In conclusion, useMemo and useCallback are powerful memoization techniques that can help optimize the performance of your React applications. By using them in components like the Counter component, you can prevent unnecessary re-renders and improve the overall performance of your application.